As the world of web development continues to evolve, the importance of APIs cannot be overstated. An API, or Application Programming Interface, is a set of protocols and tools for building software applications. REST APIs have become one of the most popular and widely used among the different types of APIs available.
This guide will cover everything you need to know about REST APIs, including their architecture, design principles, tools, and debugging techniques.
What is a REST API?
REST, or Representational State Transfer, is a set of architectural principles that define how web standards such as HTTP and URIs should be used to create scalable and flexible web services. A RESTful API is a web service that adheres to these principles.
In a RESTful API, resources are represented as URIs (Uniform Resource Identifiers) and are manipulated using standard HTTP methods such as GET
, POST
, PUT
, and DELETE
.
HTTP Verbs
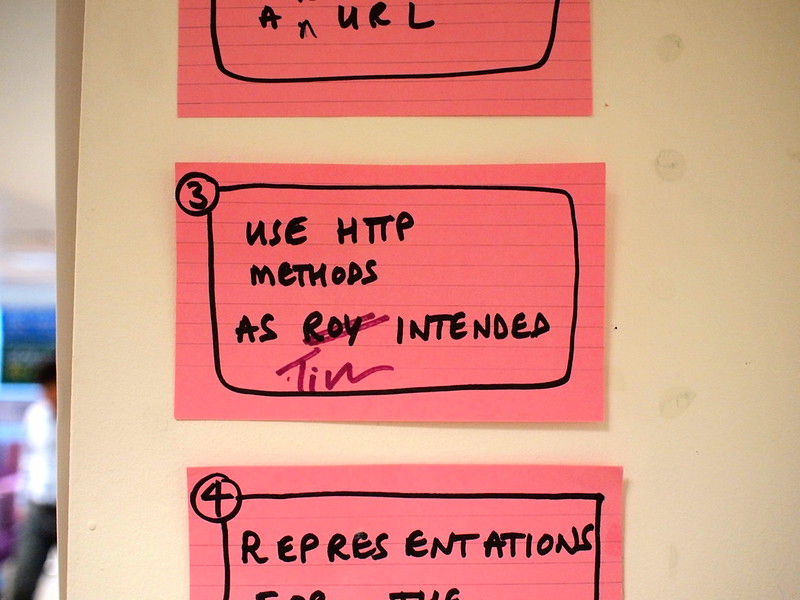
HTTP verbs are the standard methods used to interact with resources in a RESTful API. Here is a brief explanation of each of the four most commonly used HTTP verbs:
-
GET: Used to retrieve a resource from a server. For example, a
GET
request can be used to retrieve a list of all the available products on an e-commerce website. -
POST: Used to create a new resource on a server. For example, a
POST
request can be used to create a new user account on a social media website. -
PUT: Used to update an existing resource on a server. For example, a P
UT
request can be used to update a user's profile information on a social media website. -
DELETE: Used to delete a resource from a server. For example, a
DELETE
request can be used to delete a product from an e-commerce website.
HTTP Status Codes
HTTP status codes are used to indicate the success or failure of an operation in a RESTful API. Here are some of the most commonly used HTTP status codes:
-
200 OK: Indicates that the request was successful and the server returned the requested resource.
-
201 Created: Indicates that a new resource was created on the server as a result of the request.
-
400 Bad Request: Indicates that the request was malformed or contained invalid data.
-
401 Unauthorized: Indicates that the request requires authentication and the user has not provided valid credentials.
-
404 Not Found: Indicates that the requested resource could not be found on the server.
-
500 Internal Server Error: Indicates that an error occurred on the server while processing the request.
REST API Design Principles
To create a well-designed RESTful API, you should follow these principles:
-
Use HTTP methods correctly: Use GET to retrieve a resource, POST to create a new resource, PUT to update an existing resource, and DELETE to delete a resource.
-
Use URIs to identify resources: Each resource should be identified by a unique URI.
-
Use HTTP response codes correctly: Use HTTP response codes to indicate the success or failure of an operation.
-
Use consistent resource representations: Use consistent data formats, such as JSON or XML, to represent resources.
-
Use hypermedia links: Use hypermedia links to connect resources and allow clients to navigate the API.
Tools for Developing RESTful APIs
There are many tools available for developing RESTful APIs. Here are a few of the most popular ones:
-
Swagger: Swagger is an open-source framework for designing, building, documenting, and testing RESTful APIs. It provides a set of tools for creating interactive API documentation.
-
Postman: Postman is a popular API development and testing tool that allows developers to create, test, and debug APIs.
-
Insomnia: Insomnia is another API development and testing tool that offers features such as code snippets, authentication management, and automatic API testing.
-
SoapUI: SoapUI is a widely-used API testing tool that offers support for both RESTful and SOAP-based APIs.
Debugging RESTful APIs
Debugging RESTful APIs can be challenging, but there are several techniques you can use to make the process easier. Here are a few of them:
-
Use logging: Add logging statements to your code to help you understand what is happening at different points in the code.
-
Use a debugger: Use a debugger such as the one provided by your IDE to step through your code and identify problems.
-
Use a tool such as Wireshark: Wireshark is a network protocol analyzer that can help you identify issues with your API requests and responses.
-
Use an API testing tool: Use an API testing tool such as Postman or SoapUI to test your API and identify issues.
Using CURL for Testing
Here are a few examples of how to interact with a RESTful API via the command-line interface using the curl command:
- GET request:
To retrieve a resource from a server using a GET
request, you can use the following command:
curl http://example.com/api/resource
This will retrieve the resource located at the URL http://example.com/api/resource
.
- POST request:
To create a new resource on a server using a POST
request, you can use the following command:
curl -X POST -H "Content-Type: application/json" -d '{"name": "John Doe", "email": "johndoe@example.com"}' http://example.com/api/resource
This will create a new resource with the name John Doe
and email johndoe@example.com
at the URL http://example.com/api/resource
.
- PUT request:
To update an existing resource on a server using a PUT
request, you can use the following command:
curl -X PUT -H "Content-Type: application/json" -d '{"name": "Jane Doe", "email": "janedoe@example.com"}' http://example.com/api/resource/123
This will update the resource with ID 123
to have the name Jane Doe
and email janedoe@example.com
at the URL http://example.com/api/resource/123
.
- DELETE request:
To delete a resource from a server using a DELETE
request, you can use the following command:
curl -X DELETE http://example.com/api/resource/123
This will delete the resource with ID 123
from the server at the URL http://example.com/api/resource/123
.
Note that these are just basic examples and the specific syntax and parameters may vary depending on the API you are interacting with. It's always a good idea to refer to the API documentation for the correct syntax and usage of the API endpoints.
Conclusion
RESTful APIs are an essential component of modern web development. By following the design principles outlined in this guide and using the tools and debugging techniques discussed, you can create robust and reliable RESTful APIs that meet the needs of your users. Remember to use HTTP verbs and HTTP status codes correctly to ensure that your API is easy to use and understand.
Comments